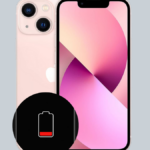
iPhone 13 mini Akku Austausch
14. August 2024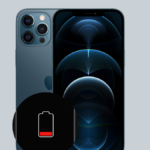
iPhone 12 Pro Max Akku Austausch
14. August 2024iPhone 13 Akku Austausch
Reparatur innerhalb von 24 Stunden
Kein Datenverlust beim Akku Austausch
Hochwertiger OEM Akku
12 Monate Garantie auf alle Reparaturen
inkl. MwSt. zzgl. Versandkosten
159,00 € Ursprünglicher Preis war: 159,00 €89,00 €Aktueller Preis ist: 89,00 €.
BESCHREIBUNG
Willkommen bei SP Poikat! Hat der Akku deines iPhone 13 an Leistung verloren? Keine Sorge, wir bieten dir einen schnellen und professionellen Akku-Tausch an! Unser erfahrenes Team ersetzt deinen alten Akku durch ein hochwertiges Ersatzteil. In kürzester Zeit ist dein iPhone 13 wieder voll einsatzbereit – mit langer Akkulaufzeit, wie am ersten Tag!
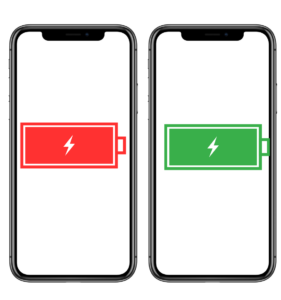
Schritt für Schritt
Formular
Kaufen Sie die gewünschte Reparaturleistung. Füllen Sie den Begleitschein vollständig aus. klick hier auf
Versenden
Sichern Sie Ihr Gerät gut und fügen Sie den ausgefüllten Begleitschein hinzu. Schicken Sie Ihr Handy an unsere DHL Lieferadresse
Reparatur
Bei Eintreffen Ihres beschädigten Smartphones führen wir sofort eine gründliche Überprüfung durch. Sie bekommen eine E-Mail von uns, sobald Ihr Handy sich wieder auf dem Rückweg zu Ihnen befindet.
Hinweis für iPhone-Nutzer:
Nach dem Austausch des Akku kann Ihr iPhone eine Fehlermeldung anzeigen. Dies ist normal und kein Grund zur Sorge. Die Meldung beeinträchtigt die Funktion Ihres Geräts nicht und ist nur eine Information des Systems. Ihr iPhone funktioniert weiterhin einwandfrei.
Ähnliche Produkte
-
iPhone 8 Plus Backcover Glas Reparatur
-
Kein Datenverlust
-
Kein öffnen Ihres Gerätes notwendig
-
Alle Farben
79,00 €Ursprünglicher Preis war: 79,00 €49,00 €Aktueller Preis ist: 49,00 €. -
-
iPhone 11 Pro Max Backcover Glas Reparatur
-
Kein Datenverlust
-
Kein öffnen Ihres Gerätes notwendig
-
Alle Farben
89,00 €Ursprünglicher Preis war: 89,00 €49,00 €Aktueller Preis ist: 49,00 €. -
-
iPhone 12 mini Backcover Glas Reparatur
-
Kein Datenverlust
-
Kein öffnen Ihres Gerätes notwendig
-
Alle Farben
99,00 €Ursprünglicher Preis war: 99,00 €59,00 €Aktueller Preis ist: 59,00 €. -
Bewertungen
Es gibt noch keine Bewertungen.